Project Background
Goal
The goal of this group assignment was to learn systems programming by creating a custom shell. The shell would run both custom commands and standard system commands.
Constraints
System()
calls could not be used — only fork()
and exec()
were allowed.
Team
I was paired with three other students. No one had programmed on a team before or used Git extensively. Only half the team — I and one other member — had working knowledge of C.
Assignment Signifigance
This is the most challenging project I've worked on, both techincally and managerially. I showcase it not because of how well it turned out, but because of how it didn't fall apart and what I learned from it.
To illustrate the techincal difficulty of this assignment, less than half the class's submissions ran without errors, even after our professor modified the syllabus — twice — to give us double the original allocated time.
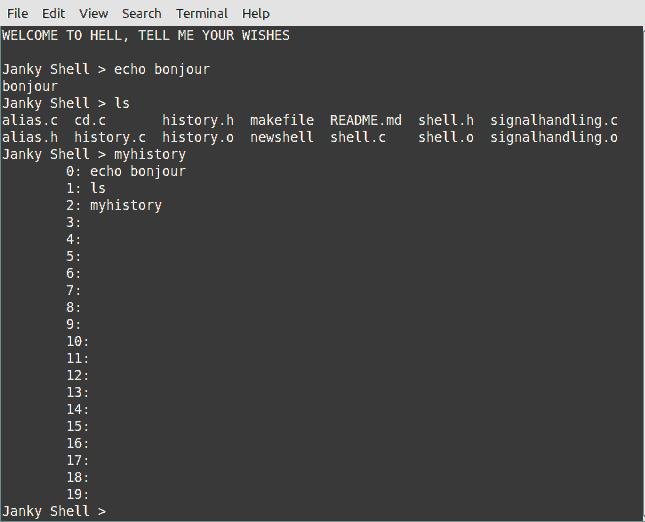
echo
, ls
) and custom (myhistory
) commands.